Functional programming is a popular software development paradigm that emphasizes immutability, modularity and avoiding side effects. In this blog, we will discuss applying functional programming with Node.js and provide code snippets to illustrate the concepts.
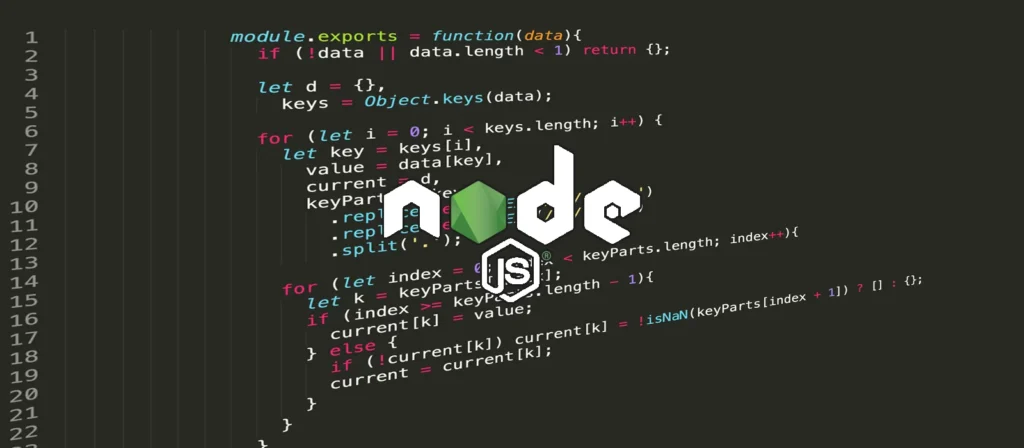
Immutability
One of the key principles of functional programming is immutability, which means that once a variable is set, it cannot be changed. In Node.js, we can achieve immutability by using the Object.freeze() method. Here’s an example:
let user = { name: 'John Doe', age: 30 };
Object.freeze(user);
// trying to change the value of a property in the frozen object
user.age = 40;
console.log(user.age); // 30
In this example, we created an object user
with properties name
and age
. We then froze the object using the Object.freeze()
method, and attempted to change the age
property. However, since the object is frozen, the value of the age
property remains unchanged.
Modularity
Another important aspect of functional programming is modularity, which involves breaking down a program into smaller, reusable pieces. In Node.js, we can achieve modularity by using functions and exporting them as modules. Here’s an example:
// greetings.js
exports.sayHello = (name) => {
return `Hello, ${name}!`;
};
exports.sayGoodbye = (name) => {
return `Goodbye, ${name}!`;
};
// index.js
const greetings = require('./greetings');
console.log(greetings.sayHello('John Doe')); // Hello, John Doe!
console.log(greetings.sayGoodbye('John Doe')); // Goodbye, John Doe!
In this example, we have two files: greetings.js
and index.js
. The greetings.js
file exports two functions, sayHello
and sayGoodbye
, which can be used to generate greetings. The index.js
file requires the greetings
module and uses the exported functions to generate greetings for the name John Doe
.
Avoiding Side Effects
Finally, functional programming emphasizes avoiding side effects, which are unintended changes to a program’s state. In Node.js, we can avoid side effects by using pure functions, which are functions that always return the same output for a given input and do not modify any external state. Here’s an example:
let user = { name: 'John Doe', age: 30 };
const addYears = (user, years) => {
return { ...user, age: user.age + years };
};
let updatedUser = addYears(user, 10);
console.log(user.age); // 30
console.log(updatedUser.age); // 40
In this example, we created an object user with properties name and age. We then defined a pure function addYears that takes an object user and a number years and returns a new object with the age property updated by the value of years. The original user object remains unchanged, and the new object is stored in the updatedUser variable.
Want to read more about javascript, we have a lot of articles helping you to fine tune your JS skills. Click here.
0 Comments